JavaScript의 Date Method의 사용 방법
JavaScript의 Date Method의 사용 방법에 대해서 알아보자.
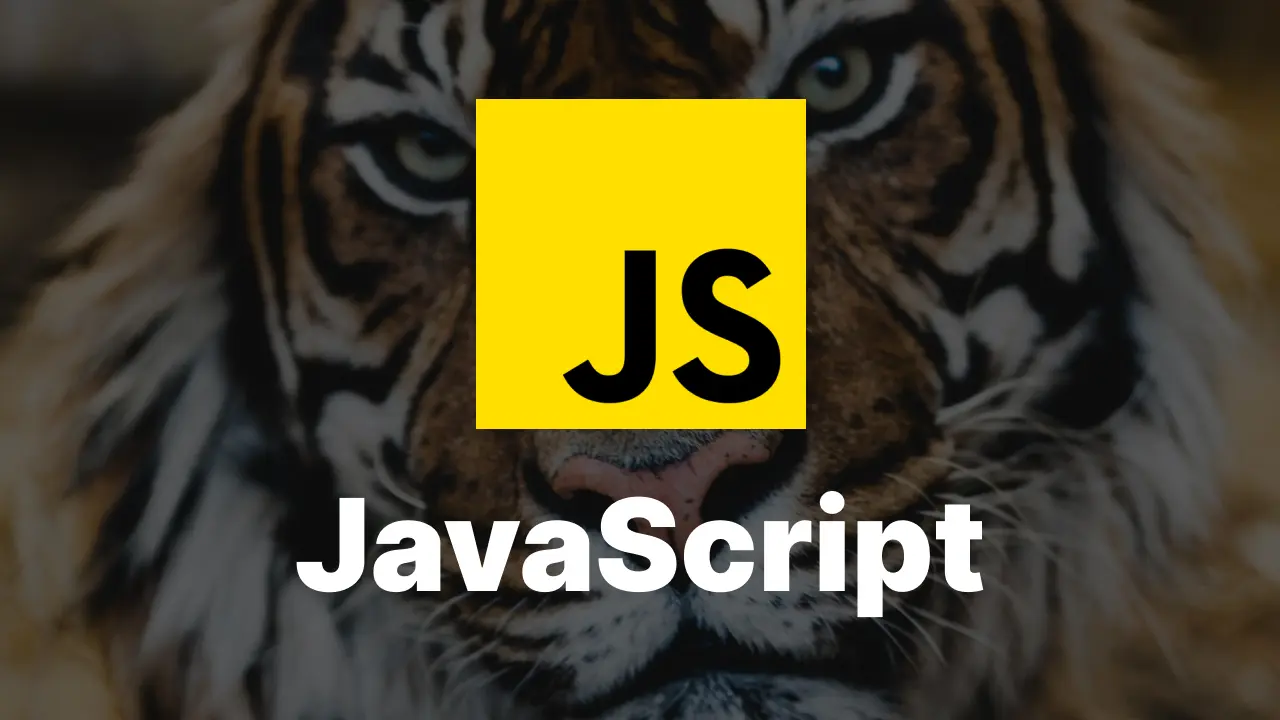
Date 객체 생성하기
JavaScript에서 날짜와 시간을 다루기 위해 Date 객체를 생성하는 방법입니다.
// 현재 날짜와 시간으로 Date 객체 생성
const now = new Date();
console.log(now);
// 예: Fri Mar 31 2025 15:30:45 GMT+0900 (한국 표준시)
// 특정 날짜로 Date 객체 생성 (연, 월(0-11), 일, 시, 분, 초)
const specificDate = new Date(2025, 2, 31, 12, 30, 0);
console.log(specificDate);
// 예: Mon Mar 31 2025 12:30:00 GMT+0900 (한국 표준시)
// 타임스탬프(밀리초)로 Date 객체 생성
const timestamp = new Date(1711939845000);
console.log(timestamp);
// 예: Sun Apr 01 2024 12:30:45 GMT+0900 (한국 표준시)
// 날짜 문자열로 Date 객체 생성
const dateString = new Date('2025-03-31T12:30:45');
console.log(dateString);
// 예: Mon Mar 31 2025 12:30:45 GMT+0900 (한국 표준시)
getFullYear()
Date 객체의 연도를 반환합니다.
const date = new Date('2025-03-31');
console.log(date.getFullYear());
// 2025
getMonth()
Date 객체의 월을 반환합니다 (0-11, 0은 1월).
const date = new Date('2025-03-31');
console.log(date.getMonth());
// 2 (3월)
getDate()
Date 객체의 일(1-31)을 반환합니다.
const date = new Date('2025-03-31');
console.log(date.getDate());
// 31
getDay()
Date 객체의 요일(0-6, 0은 일요일)을 반환합니다.
const date = new Date('2025-03-31');
console.log(date.getDay());
// 1 (월요일)
getHours()
Date 객체의 시간(0-23)을 반환합니다.
const date = new Date('2025-03-31T15:30:45');
console.log(date.getHours());
// 15
getMinutes()
Date 객체의 분(0-59)을 반환합니다.
const date = new Date('2025-03-31T15:30:45');
console.log(date.getMinutes());
// 30
getSeconds()
Date 객체의 초(0-59)를 반환합니다.
const date = new Date('2025-03-31T15:30:45');
console.log(date.getSeconds());
// 45
getMilliseconds()
Date 객체의 밀리초(0-999)를 반환합니다.
const date = new Date('2025-03-31T15:30:45.123');
console.log(date.getMilliseconds());
// 123
getTime()
1970년 1월 1일 00:00:00 UTC부터 Date 객체까지의 밀리초를 반환합니다.
const date = new Date('2025-03-31');
console.log(date.getTime());
// 1743522000000 (예시값)
setFullYear()
Date 객체의 연도를 설정합니다.
const date = new Date('2025-03-31');
date.setFullYear(2026);
console.log(date);
// 예: Tue Mar 31 2026 00:00:00 GMT+0900 (한국 표준시)
setMonth()
Date 객체의 월(0-11)을 설정합니다.
const date = new Date('2025-03-31');
date.setMonth(5); // 6월로 설정
console.log(date);
// 예: Mon Jun 30 2025 00:00:00 GMT+0900 (한국 표준시)
setDate()
Date 객체의 일(1-31)을 설정합니다.
const date = new Date('2025-03-31');
date.setDate(15);
console.log(date);
// 예: Sat Mar 15 2025 00:00:00 GMT+0900 (한국 표준시)
setHours()
Date 객체의 시간(0-23)을 설정합니다.
const date = new Date('2025-03-31T12:00:00');
date.setHours(18);
console.log(date);
// 예: Mon Mar 31 2025 18:00:00 GMT+0900 (한국 표준시)
setMinutes()
Date 객체의 분(0-59)을 설정합니다.
const date = new Date('2025-03-31T12:00:00');
date.setMinutes(30);
console.log(date);
// 예: Mon Mar 31 2025 12:30:00 GMT+0900 (한국 표준시)
setSeconds()
Date 객체의 초(0-59)를 설정합니다.
const date = new Date('2025-03-31T12:00:00');
date.setSeconds(45);
console.log(date);
// 예: Mon Mar 31 2025 12:00:45 GMT+0900 (한국 표준시)
setMilliseconds()
Date 객체의 밀리초(0-999)를 설정합니다.
const date = new Date('2025-03-31T12:00:00');
date.setMilliseconds(500);
console.log(date);
// 예: Mon Mar 31 2025 12:00:00.500 GMT+0900 (한국 표준시)
setTime()
Date 객체의 시간을 1970년 1월 1일 00:00:00 UTC부터의 밀리초로 설정합니다.
const date = new Date();
date.setTime(1743522000000);
console.log(date);
// 예: Mon Mar 31 2025 00:00:00 GMT+0900 (한국 표준시)
toDateString()
Date 객체의 날짜 부분을 사람이 읽을 수 있는 문자열로 반환합니다.
const date = new Date('2025-03-31');
console.log(date.toDateString());
// "Mon Mar 31 2025"
toTimeString()
Date 객체의 시간 부분을 사람이 읽을 수 있는 문자열로 반환합니다.
const date = new Date('2025-03-31T12:30:45');
console.log(date.toTimeString());
// "12:30:45 GMT+0900 (한국 표준시)"
toISOString()
Date 객체를 ISO 형식 문자열로 변환합니다.
const date = new Date('2025-03-31T12:30:45.123Z');
console.log(date.toISOString());
// "2025-03-31T12:30:45.123Z"
toLocaleDateString()
Date 객체의 날짜 부분을 지역화된 형식의 문자열로 반환합니다.
const date = new Date('2025-03-31');
console.log(date.toLocaleDateString('ko-KR'));
// "2025. 3. 31."
console.log(date.toLocaleDateString('en-US'));
// "3/31/2025"
toLocaleTimeString()
Date 객체의 시간 부분을 지역화된 형식의 문자열로 반환합니다.
const date = new Date('2025-03-31T12:30:45');
console.log(date.toLocaleTimeString('ko-KR'));
// "오후 12:30:45"
console.log(date.toLocaleTimeString('en-US'));
// "12:30:45 PM"
toLocaleString()
Date 객체를 지역화된 날짜와 시간 형식의 문자열로 반환합니다.
const date = new Date('2025-03-31T12:30:45');
console.log(date.toLocaleString('ko-KR'));
// "2025. 3. 31. 오후 12:30:45"
console.log(date.toLocaleString('en-US'));
// "3/31/2025, 12:30:45 PM"
날짜 계산하기
Date 객체를 사용한 날짜 계산 예시입니다.
// 날짜 더하기
const date = new Date('2025-03-31');
date.setDate(date.getDate() + 7); // 7일 후
console.log(date);
// 예: Mon Apr 07 2025 00:00:00 GMT+0900 (한국 표준시)
// 날짜 빼기
const today = new Date('2025-03-31');
const someday = new Date('2025-03-15');
const diffTime = today.getTime() - someday.getTime();
const diffDays = Math.floor(diffTime / (1000 * 60 * 60 * 24));
console.log(diffDays);
// 16
// 월 더하기
const currentDate = new Date('2025-03-31');
currentDate.setMonth(currentDate.getMonth() + 3); // 3개월 후
console.log(currentDate);
// 예: Wed Jul 01 2025 00:00:00 GMT+0900 (한국 표준시)
Date.now()
현재 시간을 1970년 1월 1일 00:00:00 UTC부터의 밀리초로 반환합니다.
console.log(Date.now());
// 1743522000000 (예시값, 현재 시간에 따라 다름)
Date.parse()
날짜 문자열을 파싱하여 1970년 1월 1일 00:00:00 UTC부터의 밀리초로 반환합니다.
console.log(Date.parse('2025-03-31'));
// 1743522000000 (예시값)
console.log(Date.parse('March 31, 2025'));
// 1743522000000 (예시값)
UTC 메소드
UTC(협정 세계시) 기준의 날짜와 시간을 다루는 메소드입니다.
const date = new Date('2025-03-31T12:30:45Z');
console.log(date.getUTCFullYear());
// 2025
console.log(date.getUTCMonth());
// 2 (3월)
console.log(date.getUTCDate());
// 31
console.log(date.getUTCHours());
// 12
요일 이름 얻기
Date 객체로부터 요일 이름을 얻는 방법입니다.
const date = new Date('2025-03-31');
const days = [
'일요일',
'월요일',
'화요일',
'수요일',
'목요일',
'금요일',
'토요일',
];
const dayName = days[date.getDay()];
console.log(dayName);
// "월요일"
월 이름 얻기
Date 객체로부터 월 이름을 얻는 방법입니다.
const date = new Date('2025-03-31');
const months = [
'1월',
'2월',
'3월',
'4월',
'5월',
'6월',
'7월',
'8월',
'9월',
'10월',
'11월',
'12월',
];
const monthName = months[date.getMonth()];
console.log(monthName);
// "3월"